 |
Lecture 15: of variables and procedures
|
|
This lecture:
-
Global and local variables
-
Passing by value, passing by reference.
Scope of variables: Global or local
Global variables
are variables that can be used everywhere in the program
|
Local variables
are only defined inside a procedure or function.
|
With this new information, it is much more clear which variable we
can use when. Inside procedures and functions we can use the global and
the local variables. Outside the procedures and functions we can only use
the global variables. Remember again what we learned in lecture12
(see the image on the right). The main program cannot use the variables
of the modules, but the other way around is allowed. |
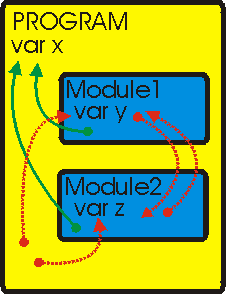 |
Warning:
try to avoid the use of global variables in procedures as much as possible.
The reason why is simple. If we want to copy the procedure for another
program this will be more difficult, becuase in the new program probably
will not have the same global variables. Using only local variables in
procedures is therefore much better. If you want to use the global variables,
pass them as parameters to the procedure. Ideally, a procedure is
a stand-alone unit. |
One more rule, typically for PASCAL and languages alike (single-pass
compilers): variables can only be used in places AFTER their declaration
in the program, so, if we put the declaration of a variable after a procedure,
this procedure cannot use the variable.
Let's take a look at some examples. First a program of lecture 13: |
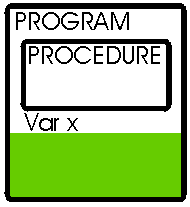 |
PROGRAM WithParameters;
(* global variables x and z *)
Var x, y: real;
FUNCTION Square(r: real): real;
(* local variable localr *)
var localr: real;
begin
localr := r*r;
x := localr;
Square := localr;
end;
begin
x := 4.0;
y := Square(x);
end.
Priority
Variable x is a local and a global variable.
Inside the procedure, the local variable
will be used.
|
When local and global variables exist with the same name, the local
variable has higher priority and will therefore be used inside the procedure.
Anyway, this is confusing, so always try to avoid using the same identifier
again!
Some languages do not have the difference between local and global variables
(for example BASIC). This will mean that we cannot use the same name for
a variable twice. |
Passing by value or by reference
When passing parameters to procedures or functions, we can do this in two
different ways, either passing by value, or passing by reference.
Passing by value:
Until now we have only seen the first type. In this way, only a value
is passed to the functions. Whatever we do with that value in the procedure
will have no effect on the original value of the variable used in calling
the procedure. As an example, to make this more clear. Assume we have a
procedure that writes the square of the parameter p. To calculate the square
we assign a new value (p*p) to p. The value of p will therefore change
inside the procedure:
PROCEDURE WriteSquare(p: real);
begin
p := p*p;
WriteLn(p:0:1);
end;
When we now call the procedure with a variable x, the value of this
variable will not change by calling the procedure. In the main program:
begin
x := 2.0;
WriteSquare(x);
WriteLn(x:0:1);
end.
After returning from the procedure, the value of x has not changed.
The total output of the program above will therefore be
4.0
2.0
Passsing by reference:
On the other hand, if we do want to change the value of the variable
used in calling the procedure, we can specify this at the definition of
the procedure by placing the word Var in front of every parameter that
we want to change permanently:
PROCEDURE WriteSquare(Var p:
real);
begin
p := p*p;
WriteLn(p:0:1);
end;
If we now run the program, the output will be
4.0
4.0
because the value of x has changed simultaneously with the value of
the parameter p.
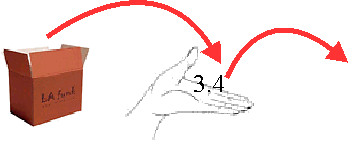 |
|
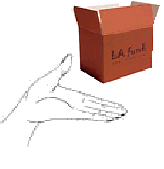 |
Passing by value
|
|
Passing by reference
|
In the analogon of boxes visualizing variables: passing by reference is
handing over the box (variable) to the procedure which can then use and
change the value in the box and at the end handing it back, while passing
by value is equivalent to opening the box, copying the value and handing
only that value over to the procedure. Obviously, then the original value
stays in the box.
Or in another example: I can tell you how much is on my bank account,
which you can then use to calculate how much it is in dollars, or I can
give you the right to change the amount on my bank account, in which case,
the amount will probably change.
Quick Test
To test your knowledge of what you have learned in this lesson, click here
for an on-line test.
Peter Stallinga. Universidade do Algarve, 5 Abril 2002