 |
Lecture 21: Files
|
|
Output to File
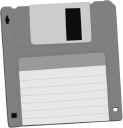
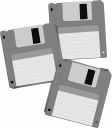
Today
we are going to learn how to read from files and write to file. As an
example
we will only learn how to read and write text files, files where the
infomation
is stored in ASCII format. Such files differ from binary format because
they are also readable by humans. We already are using with text files,
because all our programs written in the practical lessons are of this
type.
These files can be placed on floppy disks, on the harddisk, or even
on CD-ROMs (in which case they can - of course - only be read and not
written).
Instructions
The following instruction are related to file access:
Var text
Assign
Rewrite
Reset
Close
Read, ReadLn
Write, WriteLn
Eol, Eof |
Declaring a variable for file access:
Before we can open a file and have access to it (either reading or
writing)
we have to declare the existence of a file. In PASCAL we can
declare
a text file in the following way:
With filehandle the logical
name
of the file. This is not equal to the actual name of the file, as we
will
see in a moment. The place to declare this is together with the files.
Example:
Var f: text;
Assigning a name to the file
Inside the program we should assign an actual name to the file before
we
can open it:
Assign(filehandle,filename); |
The filehandle is the one
declared
above and the filename is a
string
(constant or variable) containing the name of our file. For example:
Assign(f, 'MYFILE.TXT');
ReadLn(s);
Assign(f, s);
Input or output?
To open the file, we can use two forms, depending if we want to open
the
file for input (reading the file) or output (writing to the file):
Reset(filehandle);
|
Rewrite(filehandle);
|
For example:
Reset(f);
Rewrite(f);
Reading and Writing
The reading from file and writing to file are now the same as if we
were
reading from the keyboard or writing to the screen. We use the same
instructions
(Read, ReadLn, Write, and WriteLn). The only difference is that the
first
parameter has to be the file we have just opened:
Read(filehandle,...);
|
ReadLn(filehandle,...);
|
Write(filehandle,...);
|
WriteLn(filehandle,...);
|
Note that we can only use the Read
and ReadLn instructions for
files
that have previously been opened for input and Write
and WriteLn are only to be used
for
files opened for output. Examples:
WriteLn(f, r:0:2);
ReadLn(f, opcao);
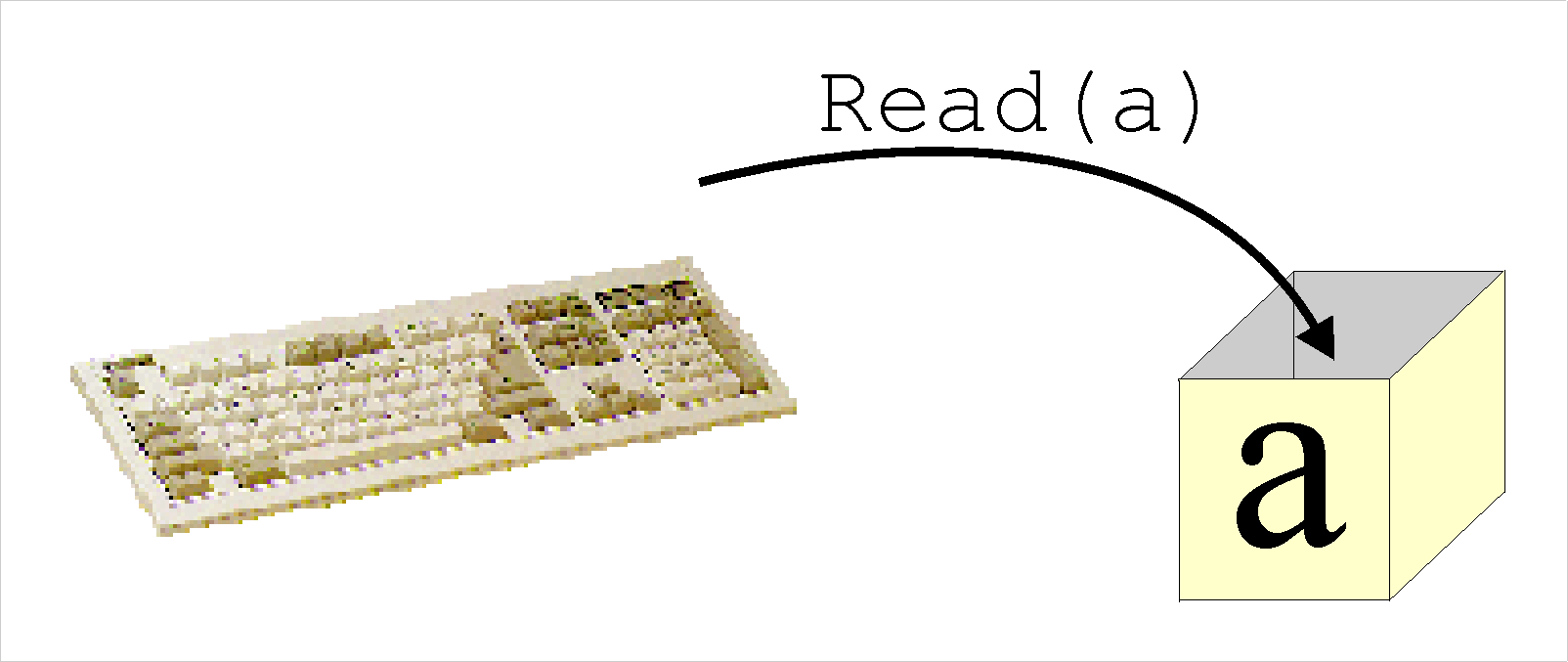 |
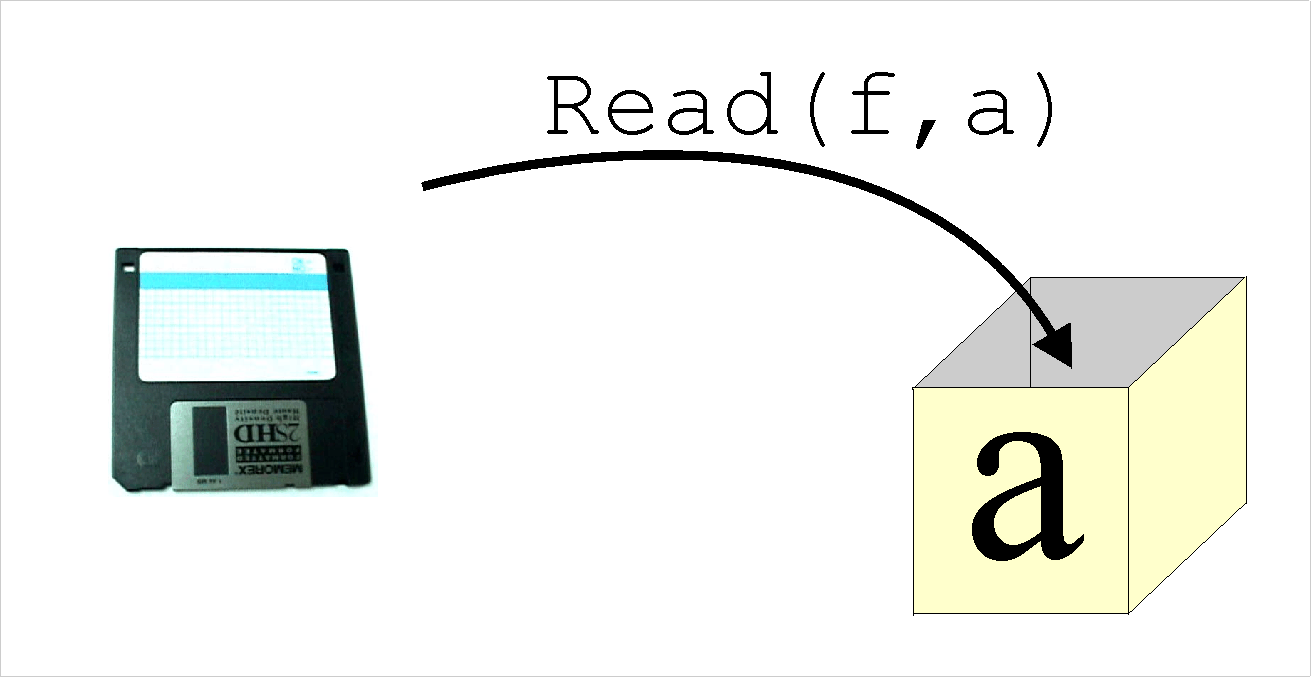 |
Read a variable with the keyboard
|
Read a variable from file
|
Closing the file
When we are ready with the file, we must close it. This is especially
the
case for output files. If we forget to close the file before ending the
program, probably not all the information will be written to the file.
To close the file we use
for example:
Close(f);
Summary
File input and output consist of the following steps:
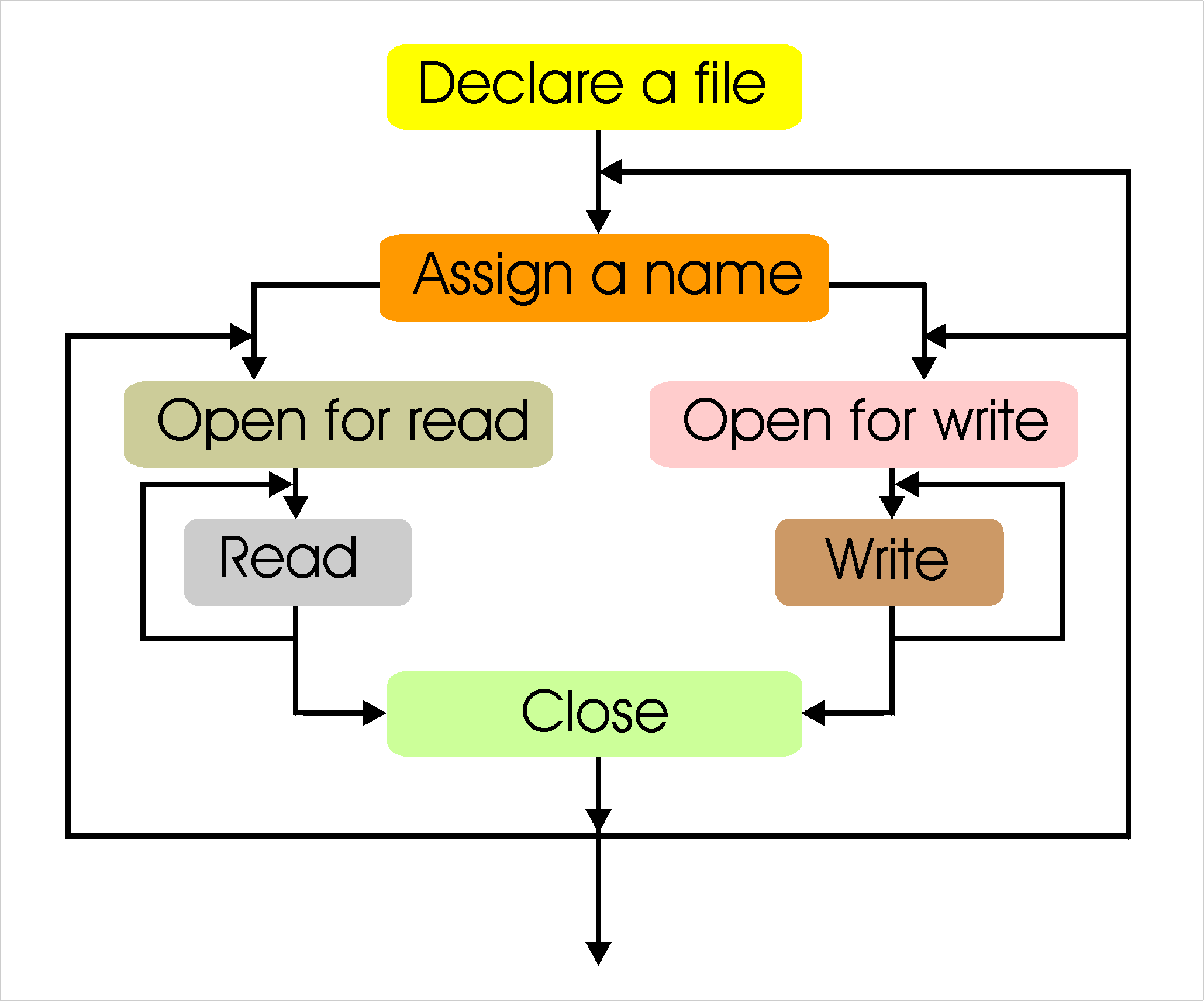
Eol, Eof
Two instructions can be useful
Eol(filehandle): returns true if we are reading
at the end of a line in the file
Eof(filehandle): returns true if we are reading
at the end of the file.
Example:
While NOT Eof(f) do
ReadLn(s);
which will read from the file until the end of
the file is encountered.
Examples
code |
screen |
file TEST.TXT
after running the program |
PROGRAM WithFileOutPut;
Var f: text;
s: string;
i: integer;
begin
WriteLn('Name of File:');
ReadLn(s);
Assign(f, s);
Rewrite(f);
for i := 1 to 10 do
WriteLn(f, i, '
Hello');
Close(f);
end.
|
Name of File:
TEST.TXT |
1 Hello
2 Hello
3 Hello
4 Hello
5 Hello
6 Hello
7 Hello
8 Hello
9 Hello
10 Hello |
code |
screen |
file TEST.TXT before
running the program |
PROGRAM WithFileInPut;
Var f: text;
c: char;
s: string;
i: integer;
begin
WriteLn('Name of File:');
ReadLn(s);
Assign(f, s);
Reset(f);
While NOT Eof(f) do
begin
(*
read
a character
from file: *)
Read(f,
c);
(*
show
it on the screen: *)
Write(c);
end;
Close(f);
end.
|
Name of File:
TEST.TXT
1 Hello
2 Hello
3 Hello
4 Hello
5 Hello
6 Hello
7 Hello
8 Hello
9 Hello
10 Hello |
1 Hello
2 Hello
3 Hello
4 Hello
5 Hello
6 Hello
7 Hello
8 Hello
9 Hello
10 Hello |
Peter Stallinga.
Universidade
do Algarve, 30 Abril 2002