 |
Lecture 4: Introduction to PASCAL
|
|
PASCAL
PASCAL is a french acronym for "Program Appliqué à
la Selection et la Compilation Automatique de la Literature" a high-level
("fourth generation") computer-programming language. Designed by Niklaus
Wirth in the 1960s as an aid to teaching programming. It is still widely
used as such in universities, and as a good general-purpose programming
language. Most professional programmers, however, now use C or C++. Pascal
was named after the 17th century French mathematician Blaise Pascal.
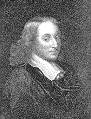 |
Blaise Pascal (1623-1662)
French philosopher and mathematician. He contributed to the development
of hydraulics,
the calculus, and the mathematical theory of probability.
His most famous invention was probably the "Pascal Triangle":
Each number is the sum of the two numbers immediately above it,
left and right, like this:
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
|
Start
A program is a sequence of instructions, or statements which
inform the computer of a specific task we want it to do.
Most modern program languages are in a very readible format, close
to English, making it easy for humans to read and write programs. This
in contrast to earlier programming languages, which were closer to things
the computer understand. See for example the assembler language (aula
2). PASCAL is the language which most resembles a natural human language
and as such is best suited for explaining the art of programming.
Every PASCAL program has the same essential format, (called the template):
PROGRAM Title;
begin
program_statement_1;
program_statement_2;
|
program_statement_n;
end.
Let's take a look at this program.
-
Every program starts with the word PROGRAM followed by the title
of the program.
-
begin defines the starting point of the program.
-
end. defines the end of the file and thus the end of the program.
Note the full stop "." at the end. This is only used for the "end" statement
at the end of the program. All other statements end with a semicolon ";"
-
The combination of "begin" and "end" is a way to group instructions so
they form a block. All the statements between "begin" and "end" are treated
by the compiler as one statement.
-
In between "begin" and "end" we can put our instructions. These instructions
can be either contain instructions that PASCAL already knows, or instructions
that we are going to define (so called procedures or functions, which we
will dsicuss later).
-
PASCAL is not case sensisitive: "Program", "PROGRAM", "program", etc, are
all the same.
Identifiers
Identifiers, as the name already says, are used for identifying
things. This can be name of programs (as above), name of procedures
and functions and names of variables and constants.
This we will see in later aulas. Like in most languages, names of identifiers
have some restrictions:
-
They should start with a letter; "PROGRAM 20hours" is not allowed.
-
Followed by any combination of letters, digits or the underscore character
"_".
-
Spaces are not allowed, nor are characters like "(", "{", "[", "%", "#",
"?", etc, except "_". The reason why this is so is that these characters
are used for other things in PASCAL. They are called reserved characters.
{ } [ ] ( ) - = + / ? < > . , ; : ' " ! @ # $ % ^ & * ~
` \ | |
-
Identifiers cannot be equal to reserved keywords of PASCAL, such
as word like "program", "integer", "begin". Note that identifiers like
"program1", or "program_" are allowed, although it is advised to avoid
such confusing names. Note: In many programming environments (like the
Turbo Pascal 7 that we will use in the practical lectures), we will notice
when we are using a reserved keyword because they will change color when
we type them in.
-
Choose your identifiers well. When a program is calculating interest rates,
call it, for example "PROGRAM InterestRates" and not "PROGRAM program1".
Although it is not an error to give a program the name "program1", it is
much more intelligent to give it a more meaningful name. This helps other
people to understand your program (or yourself when you come back to the
program after a long time).
-
The minimum length of identifiers is 1, the maximum length 255. Make use
of this possibility of long names, but also remember that also long names
can make the program unreadable. Choose a "golden middle". Which of the
following do you think is best:
r := r + a;
money := money + interest;
themoneyintheaccountofpersonwithnameJohnson
:= themoneyintheaccountofpersonwithnameJohnson + thecurrentinterestrateatthetimeofthiswriting;
-
They are not case sensitive, "i" is equal to "I", etc. To make programs
more readable, follow a convention all through your program(s). The most
often used convention is lowercase for variables and UPPERCASE for CONSTANTS.
Structured programming
The
most important thing in programming is to write clear, logical and structured
programs.
-
Use meaningful names for variables, procedures and functions.
-
Use indentation. Compare the following two programs:
PROGRAM Myprogram;begin writeln("Hello
world!");end.
and
PROGRAM MyProgram;
begin
writeln("Hello world!");
end.
Both programs do exactly the same, but the second one is much more
readible. The difference is
-
Only put one statement per line.
-
Use indent. Put (2) extra spaces in the beginning of the line every time
we are one level "deeper" in the structures.
-
Seperate blocks of text (functions and procedures) with blank lines.
-
Avoid the use of "goto label" statements. With these statements, the program
rapidly starts looking like spaghetti. Whereas in BASIC (Beginner's All-purpose
Symbolic Instruction Code) the use of the GOTO statement is nearly unavoidable,
in any itself-respecting language, the goto statement should be avoided.
-
Comment. Since PASCAL is nearly like English, the program itself should
be self-explanatory. Still, in places where the idea of the program might
not be clear to the programmer, use comments. In PASCAL comments are placed
within a set of accolades: "{" and "}". The compiler stops interpreting
the text after the first "{" and resumes compiling after it encounters
the matching "}". Therfore, any text can be placed within this context.
Alternatively, in Turbo Pascal, comments can be placed within a combination
of "(*" and "*)". Note that in that case even the accolades "{" and "}"
are skipped by the compiler.
-
Use procedures and functions wherever it makes the text more organized.
If at many different places the program has to do basically the same thing
(for instance reading a line of text form a file), consider putting it
in a procedure or function (for example PROCEDURE FileReadLn;). This will
make the program more readable, more efficient and shorter.
Reserved keywords in Turbo Pascal
The following words cannot be used for identifiers. Most of these keyword
are explained in the lectures in the chapters described by the subject
in the second column.
keyword
|
subject
|
and |
boolean algebra |
asm |
|
array |
arrays |
begin |
introduction |
case |
if .. then |
const |
variables and constants |
constructor |
|
destructor |
|
div |
algebra |
do |
loops |
downto |
loops |
else |
if .. then |
end |
introduction |
exports |
|
file |
|
for |
loops |
function |
procedures and functions |
|
keyword
|
subject
|
goto |
|
if |
if .. then |
implementation |
|
in |
|
inherited |
|
inline |
|
interface |
|
label |
|
library |
|
mod |
algebra |
nil |
pointers |
not |
boolean algebra |
object |
|
of |
if .. then |
or |
boolean algebra |
packed |
|
procedure |
procedures and fucntions |
|
keyword
|
subject
|
program |
introduction |
record |
records |
repeat |
loops |
set |
|
shl |
|
shr |
|
string |
variables |
then |
if .. then |
to |
loops |
type |
variables |
unit |
|
until |
loops |
uses |
|
var |
variables |
while |
loops |
with |
records |
xor |
boolean algebra |
|
The following words are related to variables and constants. Use of these
words for identifiers is disadvised:
boolean |
byte |
char |
double |
integer |
real |
string |
text |
word |
|
|
Quick test:
To test your knowledge of what you have learned in this lesson, click
here for an on-line test. Note that this NOT the form the final
test takes!
Peter Stallinga. Universidade do Algarve, 17 fevereiro 2002