 |
Lecture 10: Loops II: while ... and do ...
while
|
|
while ...
The loops in this lecture, while and do-while are used for repeating
things
that are not exactly countable. Normally we use this when it is not
exactly
clear when the loop will finish, for instance because the control
variable
changes within the loop (as is strictly disadvised in for loops). Also,
when we want to loop over something with a floating point type variable
we use the while and do-while loops.
As we will show, the difference between the while and the do-while
loops is the moment the condition is tested.
The general format of the while loop is
while (condition)
Instruction; |
|
|
|
This structure is repeating the instruction as long as the condition is
true. The condition is any
condition
that results in a boolean value (TRUE or FALSE), as we have discussed
in
lecture
8. This can be a comparison, or anything else (still information in
file?, user pressed a key?, etc).
Note that the structure while does not attribute a starting value to
any variable like in the for-loop. So, we have to do this ourselves.
Example:
program code
main()
// while example
{
float x;
x = 0.0;
while (x<=1.0)
{
printf("%0.1f\n",
x);
x = x +
0.1;
}
}
|
output
0.0
0.1
0.2
0.3
0.4
0.5
0.6
0.7
0.8
0.9
1.0
|
do ... while
The do-while structure is very similar to the while structure. Theonly
difference is that now the condition is checked at the end of the
instructions
to be repeated. The general format is
do
Instruction;
while (condition); |
|
|
|
A very important difference between while and do-while is that with
while
the condition is checked in the beginning of the loop, whereas
in
do-while it is checked at the end. Therefore,
the instructions
in do-while are at least executed once.
Look at the following programs (also an example with a for-loop is
included). Only the code with the do-while structure has output.
program code
x = 100;
do
{
printf("Ajax\n");
x = x + 1;
}
while (x<=10); |
program code
x = 100;
while (x<=10)
{
printf("Ajax\n");
x = x + 1;
} |
program code
for (i=100; i<=10;
i++)
printf("Ajax\n"); |
output
Ajax |
output
|
output
|
for, while e do-while compared
Effectively, the for and while loops are exactly the same. Both have a
condition that is checked in the beginning of the loop and a step
instruction.
The only difference is that the for-loop already includes a starting
instruction.
If we precede the while-loop with a starting instruction, the two loops
are equal:
for
for (startI; testC;
stepI)
instruction; |
while
startI;
while (testC)
{
instruction;
stepI;
} |
for (i=1; i<=10;
i++)
printf("%d", i);
|
i=1;
while (i<=10)
{
printf("%d", i);
i++;
}
|
Only for the sake of readability of the program will we use the
for-loop
for repeating things in a countable way. For all other types of loop
will
we use while and do-while. In the do-while loop the instructions are at
least executed once. Always take these considerations into account when
deciding for the type of loop to use.
Nesting II
Now that we know all the types of loops, let's take a look at the
rules-of-good-behavior
related to nesting:
- Each (for) loop must use its own seperate control variable.
- The inner loop must begin and end entirely within the outer loop
Examples of bad code:
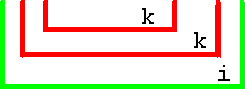 |
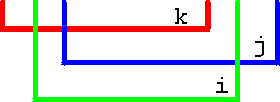 |
for (i=1; i<=10; i++)
{
for (i=1; i<=20;
i++)
printf("%d",
i);
} |
for (i=1; i<=10; i++)
{
x = i;
do
x =
x+0.1;
}
while (x<20); |
Two loops with equal control variable i. |
Two loops not well nested.
Good indentation of your program will
always avoid such errors. |
Quick test:
To test your knowledge of what you have learned in this lesson, click here
for an on-line test.
Peter Stallinga. Universidade do Algarve, 4
November
2002